Good day, I need help with this code, please.
Code:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <float.h>
#define EPS 1e-5
double gcd(double val1, double val2)
{
double tmp, a;
if(val1 > val2){
tmp = val1;
val1 = val2;
val2 = tmp;
}
a = fmod(val2, val1);
if(fabs(a) < EPS){
return val1;
}
else {
return gcd(a, val1);
}
}
double lcm(double num1, double num2)
{
double lcmr, gcdr;
gcdr = gcd(num1, num2);
lcmr = ((num1 * num2) / gcdr);
return lcmr;
}
double decimal(double a1)
{
int a1i, k;
double r, i;
k = 0;
a1i = a1;
r = (a1 - a1i);
for(i = 0; i < 1; i = i + 0.1)
{
if( fabs(i - r) <= EPS * fabs(i + r) )
{
k = 1;
}
}
if(k != 1){
printf("Nespravny vstup.\n");
return 0;
}
return k;
}
int main()
{
double tile, joint, value1, x, y, max, alf, blf;
int in, a, b;
value1 = 0.1;
max = 10000000;
printf("Minimalni velikost: \n");
if (scanf(" %lf %lf", &x, &y) !=2 || (x - max) > EPS * fabs(x + max) || (y - max) > EPS * fabs(y + max) || x <= 0 || y <= 0){
printf("Nespravny vstup.\n");
return 0;
}
decimal(x);
decimal(y);
printf("Zadej cisla: \n");
while( ( in = scanf("%lf %lf", &tile, &joint)) != EOF)
{
decimal(tile);
decimal(joint);
if(in != 2 || tile < 0 || joint < 0)
{
printf("Nespravny vstup.\n");
return 0;
}
if((tile - max) > EPS * fabs(tile + max) || tile <= 0)
{
printf("Nespravny vstup.\n");
return 0;
}
value1 = lcm(value1, tile + joint);
}
a = x / value1;
b = y / value1;
alf = a * value1;
blf = b * value1;
while(x >= alf)
{
alf = alf + value1;
}
while(y >= blf)
{
blf = blf + value1;
}
printf("alf: %lf\n", alf);
printf("blf: %lf\n", blf);
return 0;
}
I have this code and need to calculate minimal width and length of the kitchen.
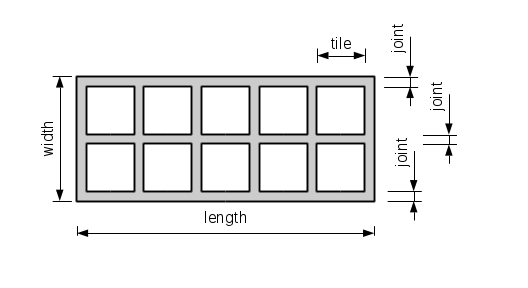
I have unknown count of tiles with unknow extent and joint. This code is perfect when joint = 0, but when I add joint, code doesnt work. The accuracy is on millimeters, so 0.1, 0.2, 0.3 ... I dont know which tile will be used so every tile + joint have to pave the kitchen. And I can use only whole tile. I just dont know, where to add the joint. Thank you.