Hi there,
I'm not sure if this belongs here or in the Gaming forum. I'll post it here as I guess a lot of the problem is C++ related. Please move it elsewhere if this is the wrong place thankyou.
Well...to begin with, when I fired up my old program I've used so many times before it suddenly ended with a bad looking error.

Then when I ran debug I ended up all the way into here:
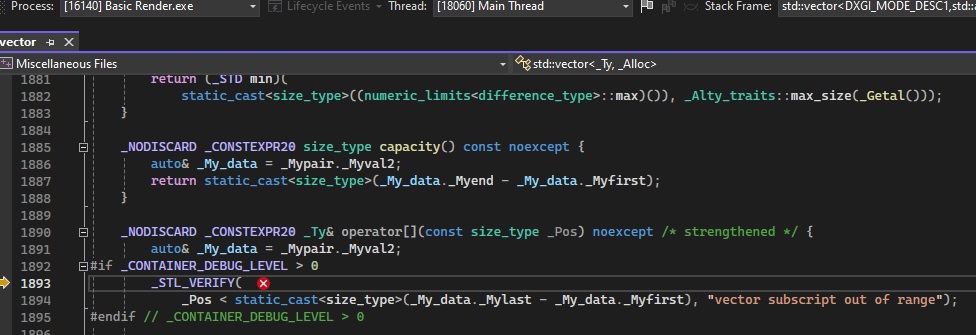
Pretty scary. Turns out a vector wasn't initialised properly. By now I'm obviously becoming worried that I will never fathom code no matter what and all sorts of thoughts are running through my mind. This is much worse if it happens with something you've made no changes to that used to work.
Well I followed the trail and found this vector was initialised to a length of 0 and then when an attempt was made to access its first element that's what generated the error:
Code:
//
std::vector<DXGI_MODE_DESC> modeList(count);
ThrowIfFailed(output->GetDisplayModeList(mBackBufferFormat, flags, &count, &modeList[0]));
//
The ThrowIfFailed macro shows detailed output relating to DirectX specific faults. I'm not actually sure this function returns a FAIL HRESULT, I just set the condition to always be true so I could see the output.
Without the original fault being there anymore I'm struggling to recreate it however it essentially said something like "A resource is not currently available but may become available later". I tried all sorts of Back Buffer Formats and flag combinations and nothing would work. Suspecting the latest Windows update had damaged the driver I set about uninstalling the card and then re-installing the manufacturer's driver rather than using device manager to do it.
I had exactly this problem when I installed the card (I'm using a very old one) in the first place. The usual nonsense of powering off, switching display cables from card to on-board, and then changing settings in the BIOS followed. I eventually got the card uninstalled and then re-installed with a installer I keep on my hard drive from AMD. To make sure, I removed the AMD driver stuff and restarted before re-installing it.
Prior to this I had even tried changing the function calls to later version in the hope that would fix it.
Code:
IDXGIAdapter1* adapter1 = nullptr;
IDXGIOutput1* output1 = nullptr;
mResolutionPair.clear();
if ((mdxgiFactory->EnumAdapters1((UINT)0, &adapter1)) != DXGI_ERROR_NOT_FOUND)
{
if ((adapter1->EnumOutputs((UINT)0, reinterpret_cast<IDXGIOutput**>(&output1)) != DXGI_ERROR_NOT_FOUND))
{
DXGI_OUTPUT_DESC desc;
output1->GetDesc(&desc);
}
}
// Call with nullptr to get list count.
ThrowIfFailed(output1->GetDisplayModeList1(mBackBufferFormat, flags, &count, nullptr));
// Check to make sure count variable is valid.
if (count == 0) { MessageBoxW(NULL, L"The variable 'count' has not been initialised correctly.", L"Error", 0); }
std::vector<DXGI_MODE_DESC1> modeList(count);
ThrowIfFailed(output1->GetDisplayModeList1(mBackBufferFormat, flags, &count, &modeList[0]));
Note the 1's at the end of the function names and the need to do a reinterpret_cast as an IDXGIAdapter1 has no member function named EnumOutputs1 and neither does its parent class IDXGIAdapter. EnumOutputs will only tolerate an argument of IDXGIOutput** not IDXGIOutput1**.
Anyways.. having updated the functions and still no luck that's when I realised it might be the driver after the latest Windows update.
This is why I always dread Windows updates 
And that is what actually fixed it. Very confusing at first. But that's all it was, a driver issue causing the function that requests the number of display modes for a specific back buffer format to fail. Hence causing a vector to be initialised as empty and then throwing a massive error when an attempt was made to access its first element.
In the code above it was the variable 'count' that was the problem. I thought I'd post this somewhat as a matter of interest to anyone it may concern but also in the hope anyone having the same problem can see what may be causing it.
Thanks