I have written a program that does matrix multiplication as in the example below and which gives me correct results (compared to online calculators).
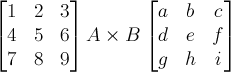
Code:
int A[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int B[3][3] = {
{a, b, c},
{d, e, f},
{g, h, i}
};
// multiplication along the lines of
// (1 * a) + (2 * d) + (3 * g)
C[0][0] = A[0][0] * B[0][0] + A[0][1] * B[1][0] + A[0][2] * B[2][0];
...
However I'm a little unsure whether I can write matrices that I find in math texts in the order in which I see them.
Taking this matrix for right-handed, CCW rotation around the x-axis for example:
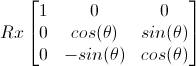
Can i write this as
Code:
// c, s are symbolic
double Rx[3][3] = {
{1, 0, 0},
{0, c, s},
{0,-s, c}
};
Or do the C++ rows represent the columns in the picture above, such that the definition becomes:
Code:
double Rx[3][3] = {
{1, 0, 0},
{0, c,-s},
{0, s, c}
};
To summarize, I'm unsure whether matrices, they way they are written in math texts, can simply be written into C++ arrays "as they appear".