Thanks. I have to pay more attention in those parts. I don't have any more segmentation fault but output is still not correct, it's printing some weird characters after the correct ones. Like barack obama gives this:
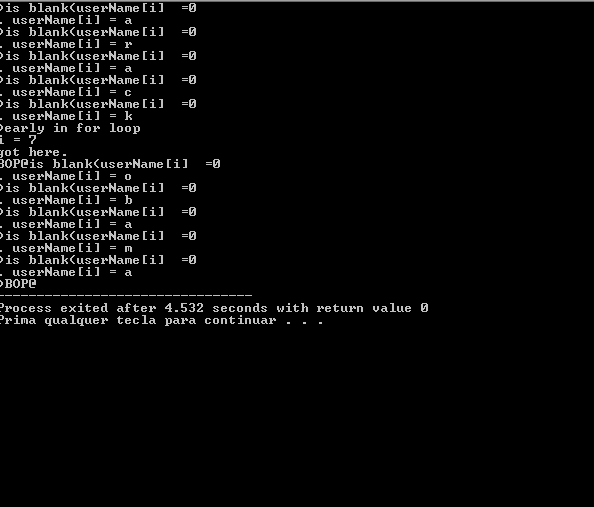
Code:
#include <stdio.h>#include <ctype.h>
#define NAME_LEN 80+1
int main()
{
char userName[NAME_LEN], newArray[NAME_LEN];
int pos = -1;
printf("Enter name: ");
fgets(userName, NAME_LEN, stdin);
printf("%s", userName);
for(int i = 0; userName[i] != '\n' && userName[i] != '\0'; i++)
{
printf("early in for loop\n");
printf("i = %d\n", i);
if (userName[i] >= 'a' && userName[i] <= 'z')
{
printf("got here.\n");
pos++;
newArray[pos] = userName[i] - ('a' - 'A');
printf("%s", newArray);
}
else
{
pos++;
newArray[pos] = userName[i];
}
while (!isblank(userName[i]) && userName[i] != '\n')
{
printf("is blank(userName[i] =%d\n, userName[i] = %c\n)", isblank(userName[i]), userName[i]);
i++;
}
}
pos++;
userName[pos] = '\0';
printf("%s", newArray);
}
Sorry for the prints, iam still in the process of learning how to use gdb, it is a bit intimidating as of now .