I'm having a little bit of trouble on an assignment and am looking for some advice. I'm supposed to create a "game" that is similar to Candy Crush or Bejeweled. The assignment takes in a .txt file that contains a matrix of values from 1-5 and then assigns each value to a spot in a [10][10] array. Then an Escape Code function prints out colored pixels in place of the number values for each spot, creating a "game board" looking output. Finally, the program is supposed to look for any matches of 3 same-colored pixels and replace them with a white pixel and "XX". Then the program prints the corrected game board with the matches X'd out.
I have it mostly coded, but I've encountered a couple of issues.
1.) I am supposed to label the columns and rows 0-9, and while I have no problem coding the labels for the columns using printf( ), when I try to print the row labels I get a random string of numbers.
2.) I replaced the matches with white pixels by reassigning the value in the array to 7, for which the ANSI Escape Code is white. However, I'm unsure about how to print the "XX" in the same spot.
Here is the input(.txt) file and the output of the program so far:

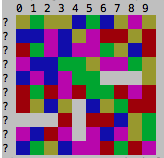
And here is what I have coded at this point:
Code:
#include <stdio.h>
void printEscapeCode(int c);
int main(void)
{
/* Declare an image array to be gameboard */
int gameboard[10][10];
/* Declare variables and load in how many rows and columns */
int Nrows;
Nrows = 0;
int Ncols;
Ncols = 0;
scanf("%d %d",&Nrows, &Ncols);
/* Load in candy values for each row */
int row, col;
for(row = 0; row < Nrows; row++)
{
/* Load in candy values for each column */
for(col = 0; col < Ncols; col++)
{
/* Declare variable to hold value */
int x;
scanf("%d",&x);
/* Tell where to store candy value */
gameboard[row][col] = x;
}
}
/* Calls function to print candy colors for each row */
printf(" 0 1 2 3 4 5 6 7 8 9\n");
for(row = 0; row < Nrows; row++)
{
printf("? ");
/* Calls function to print candy colors for each column */
for(col = 0; col < Ncols; col++)
{
if(gameboard[row][col] == gameboard[row+1][col] && gameboard[row+1][col] == gameboard[row+2][col])
{
gameboard[row][col] = 7;
gameboard[row+1][col] = 7;
gameboard[row+2][col] = 7;
}
if(gameboard[row][col] == gameboard[row][col+1] && gameboard[row][col+1] == gameboard[row][col+2])
{
gameboard[row][col] = 7;
gameboard[row][col+1] = 7;
gameboard[row][col+2] = 7;
}
if(gameboard[row][col] == 7)
{
printEscapeCode(7);
}
if(gameboard[row][col] < 7)
{
printEscapeCode(gameboard[row][col]);
}
}
printEscapeCode(7);
printf("\n");
}
return 0;
}
/* Function that prints candy colors */
void printEscapeCode(int c){
printf("\x1b[4%dm ",c);
}