Hello guys, I'm wondering if my code is correct for my assignment. I'm not to sure on this whole pointer thing at the moment.
This is my assignment
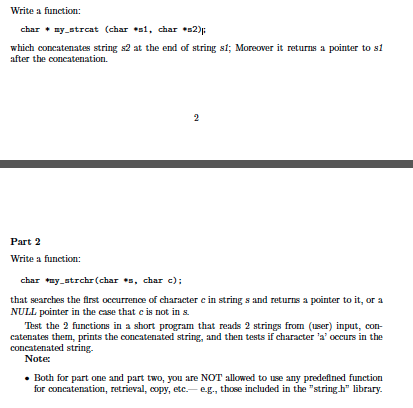
Code:
#include <stdio.h>
char *my_strcat(char *string1, char *string2)
{
int i, j;
for(i=0; string1[i]!='\0'; ++i);
for(j=0; string2[j]!='\0'; ++j, ++i)
{
string1[i]=string2[j];
}
string1[i]='\0';
return 0;
}
char *my_strchr(char *string1, char c)
{
int i, countNumberofTimes;
countNumberofTimes=0;
for(i=0 ; string1[i]!='\0' ; i++)
if(string1[i]==c)
countNumberofTimes++;
printf("The number of occurences is: %i\n",countNumberofTimes);
return 0;
}
int main()
{
char string1[200];
char string2[200];
char c;
printf("Enter the first word: ");
scanf("%s",string1);
printf("Enter the second word: ");
scanf("%s",string2);
my_strcat(string1, string2);
printf("After the concetation function this is value: %s\n",string1);
printf("Enter a Character that you'd like to be counted: ");
scanf(" %c",&c);
my_strchr(string1, c);
return 0;
}