I'm having a slight problem with my program. It keeps showing two answers and it's only supposed to show one.
Code:
#include <stdio.h>
#include <math.h>
int main()
{
float math(float x, float y);
float math2(float x, float y);
float x;
float y;
printf("Please enter a x value: ");
scanf("%d", &x);
printf("Please enter a y value: ");
scanf("%d", &y);
if(0 <= x <10)
{
printf("The result is %f.\n", math(x,y));
}
else if(x >=10)
{
printf("The result is %f.\n", math2(x,y));
}
if( y ==0)
{
printf("Error: Division by 0!");
}
return(0);
}
float math(float x, float y)
{
return (x+1)/y;
}
float math2(float x, float y)
{
return (x-9)/y;
}
This is what I get on command prompt after i enter values
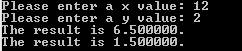
The 1.5 is correct and the answer is correct. I just can't seem to stop the first one from appearing
These are the instructions