Hi, any help would be greatly appreciated. I apologize that it's a bit sloppy. I'm having trouble understanding exactly why PS35, the last struct jobQueue processName is continually repeating when attempting to output entire queue. Is anyone able to understand what's going on?
Code:
File Input:
PS1 90001 100 90500 100
PS2 90010 50 90400 0
PS3 90011 40 90520 0
PS4 90050 300 91000 100
PS5 90065 150 90765 100
PS6 90070 500 90170 200
PS7 90080 100 91100 100
PS8 90090 150 90690 200
PS9 90167 130 91500 0
PS10 90170 500 93500 100
PS11 90172 50 90900 0
PS12 90180 105 92190 0
PS13 90190 99 93200 0
PS14 90195 475 91300 100
PS15 90200 100 91900 0
PS16 91000 130 93100 100
PS17 91001 150 93200 0
PS18 91002 350 93800 200
PS19 91050 399 93999 200
PS20 91100 300 94000 100
PS21 91110 100 91900 200
PS22 91215 500 92125 200
PS23 91235 150 92500 200
PS24 91240 300 92600 0
PS25 91320 500 93700 0
PS26 91350 700 94500 100
PS27 91630 170 92630 200
PS28 91999 119 93999 100
PS29 92001 240 94001 0
PS30 92019 191 94900 0
PS31 92201 110 94950 0
PS32 92299 299 95000 100
PS33 92301 199 95010 0
PS34 92319 100 95700 200
PS35 93001 200 96000 100
Code:
File Output:
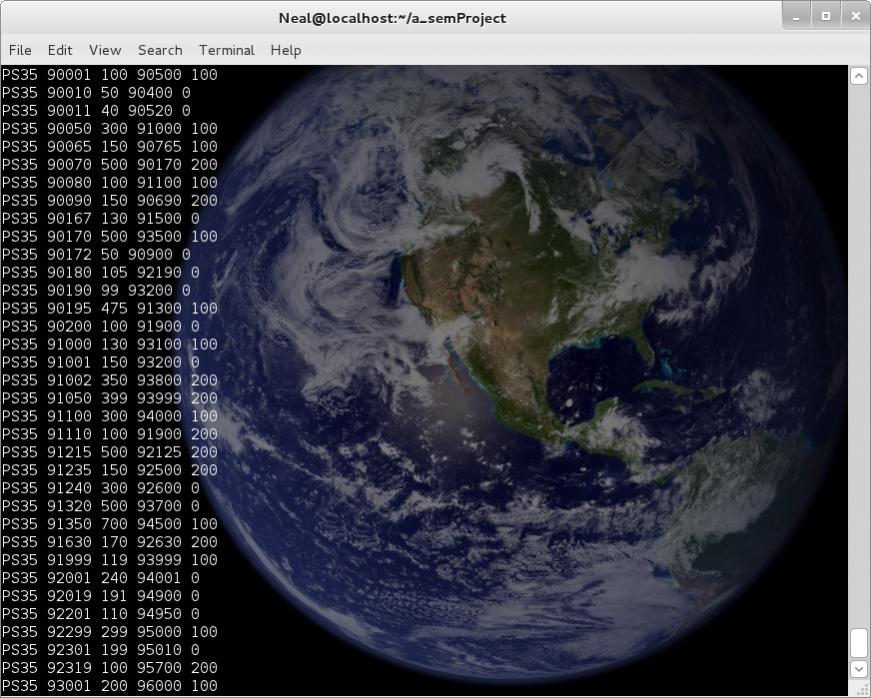
Code:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
struct dllist{
char *processName; // Length = 10
unsigned int arrivalTime; // When it processor in Ready Queue
unsigned int jobLength; // Burst Time
unsigned int deadline; // Processing Limit
unsigned int ioTime; // Time for IO = 200 x I/O Request Time
struct dllist *next;
struct dllist *prev;
};
struct dllist *head, *tail;
void append_node(struct dllist *lnode);
void insert_node(struct dllist *lnode, struct dllist *after);
void remove_node(struct dllist *lnode);
void import_file(char *);
int main()
{
import_file("testFile.txt");
return 0;
}
void import_file(char *fileName){
struct dllist *jobQueue;
FILE *fp;
char buf[100];
fp = fopen(fileName,"r");
if(!fp)
{
printf("%s\n","Input_2012.txt: File not found");
}
while(fgets(buf,100,fp)!=NULL) // Gets from file each 100 bit line.
{
char *pch; // Stores current token
char *temp_pch;
unsigned int temp_data;
jobQueue = (struct dllist *)malloc(sizeof(struct dllist));
pch = strtok(buf," ,.-\t");
temp_pch = pch;
jobQueue->processName = temp_pch;
pch = strtok(NULL," ,.-\t");
temp_pch = pch;
temp_data = (unsigned int) atoi(temp_pch);
jobQueue->arrivalTime = temp_data;
pch = strtok(NULL," ,.-\t");
temp_pch = pch;
temp_data = (unsigned int) atoi(temp_pch);
jobQueue->jobLength = temp_data;
pch = strtok(NULL," ,.-\t");
temp_pch = pch;
temp_data = (unsigned int) atoi(temp_pch);
jobQueue->deadline = temp_data;
pch = strtok(NULL," ,.-\t");
temp_pch = pch;
temp_data = (unsigned int) atoi(temp_pch);
jobQueue->ioTime = temp_data;
append_node(jobQueue);
}
fclose(fp);
struct dllist *jq;
for(jq = head; jq != NULL; jq = jq->next) {
printf("%s ", jq->processName);
printf("%d ", jq->arrivalTime);
printf("%d ", jq->jobLength);
printf("%d ", jq->deadline);
printf("%d\n", jq->ioTime);
}
}
void append_node(struct dllist *lnode)
{
if(head == NULL)
{
head = lnode;
lnode->prev = NULL;
}
else
{
tail->next = lnode;
lnode->prev = tail;
}
tail = lnode;
lnode->next = NULL;
}
void insert_node(struct dllist *lnode, struct dllist *after)
{
lnode->next = after->next;
lnode->prev = after;
if(after->next != NULL)
after->next->prev = lnode;
else
tail = lnode;
after->next = lnode;
}
void remove_node(struct dllist *lnode)
{
if(lnode->prev == NULL)
head = lnode->next;
else
lnode->prev->next = lnode->next;
if(lnode->next == NULL)
tail = lnode->prev;
else
lnode->next->prev = lnode->prev;
}