Hi, everyone. I was studying a C program that applies the ++ and * operatores on pointers, but I don't understand the output. The code is:
Code:
#include <stdio.h>
int data[2] = {100, 200};
int moredata[2] = {300, 400};
int main(void)
{
int * p1, * p2, * p3; //declaration of three pointers
p1 = p2 = data; //p1 = p2 = &data[0]
p3 = moredata; //p3 = &moredata[0]
printf(" *p1 = %d, *p2 = %d, *p3 = %d\n", *p1, *p2, *p3);
printf("*p1++ = %d, *++p2 = %d, (*p3)++ = %d\n", *p1++, *++p2, (*p3)++);
printf(" *p1 = %d, *p2 = %d, *p3 = %d\n", *p1, *p2, *p3);
return 0;
}
The output is:
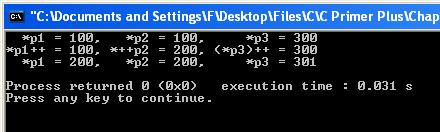
How come *p1++ is still 100? The way I see it, first p1 is incremented, poiting to data[1], and then the * operator should get 200 instead of 100. But this value is only obtained in the following printf line. And why is (*p3)++ 300 and not 301?
Thanks in advance!