hi,
I wrote a code to check the endianess of my PC. this is a core I-5, so 8086 based
processor, should be little endian.I am using Visual Studio 2010.
The data i have is 0x0100 or 256d. in little endian, lower address should store
LSByte or 0x00 and higher address should have MSbyte as 0x01. that i verified
by the code below.
Code:
#include <stdio.h>
int main()
{
short int a= 256; // 0x0100
unsigned char *p = (unsigned char *)&a;
unsigned char ch[3] = "AB"; //0x410x42
unsigned char *p1= ch;
printf (" *p= %x and *(p+1)=%x\n ", *p,*(p+1));
a= a<<7; // to confirm the little endian memory organization
printf (" *p= %x and *(p+1)=%x\n ", *p,*(p+1));
//** repeat for the string**//
printf (" *p1= %x and *(p1+1)=%x\n ", *p1,*(p1+1));
ch[0]= ch[0]<<7;
ch[1]= ch[1]<<7;
printf (" *p1= %x and *(p1+1)=%x\n ", *p1,*(p1+1));
}
I have following questions
1) as seen in the attachment, memory at address 0x0033FEA4 (of variable a) shows 0x0100 which means address that grows from left to right. is this the way its actually stored in the memory?or it is that variable a is stored other way round but Visual Studio shows it like this
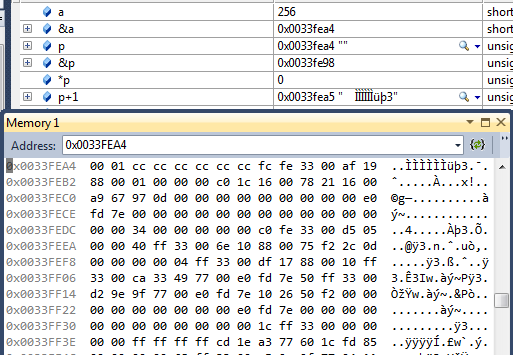
so its just a different visualization but not actual hardware memory organization?
2) in case of string ch= "AB" which is 0x41 and 0x42, following the same analogy,
ch[0] or lower address in little endianness should have LSB as 'B' or 0x42 , and higher address should have MSB as 'A' or 0x41. however, what is see in the memory window is opposite as shown below
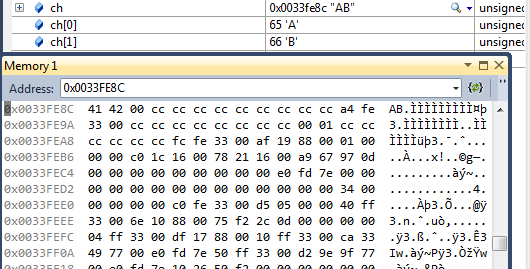
why?
I found a reference to this in the wikipedia article at
http://en.wikipedia.org/wiki/Endiann
ess and is shown in figure 3.

these results are also given in the output window whose snapshot is given in figure 4.

thanks
sedy