Code:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <time.h>
int xPosCalc(int sigmaMatter, int muMatter, int sigmaAntimatter, int muAntimatter, int xRand)
{
int seed = time(NULL);
srand(seed);
xRand = rand() % 36;
int xPosMatter = 1/(sigmaMatter*sqrt(2*3.14159))*exp(-((xRand-muMatter)*(xRand-muMatter))/(2*(sigmaMatter*sigmaMatter)));
int xPosAntimatter = 1/(sigmaAntimatter*sqrt(2*3.14159))*exp(-pow((xRand-muAntimatter), 2)/(2*(pow(sigmaAntimatter, 2))));
int xProbability = xPosMatter * xPosAntimatter;
//printf("\nMatter: %d\nAntimatter: %d", xPosMatter, xPosAntimatter);
//printf("\nProbability is: %d", xProbability);
}
int main(void)
{
int sigmaMatter = 4.2;
int muMatter = 27.0;
int sigmaAntimatter = 6.0;
int muAntimatter = 17.0;
int xRand = 0.0;
xPosCalc(sigmaMatter, muMatter, sigmaAntimatter, muAntimatter, xRand);
int i, p;
int count = 0;
int pTotal = 0;
for(i =0; i <= 4999; i=i+1)
{
p = 1/(4.2*sqrt(2*3.14159))*exp(-((27-xRand)*(27-xRand))/((2*4.2)*(2*4.2)))*1/(6*sqrt(2*3.14159))*exp(-((17-xRand)*(17-xRand))/((2*6)*(2*6)));
count++;
pTotal = pTotal + p;
}
int pFinal = pTotal/count;
printf("\npTotal: %d", pTotal);
printf("\nTotal iterations: %d", count);
printf("\nFinal probability: %d\n", pFinal);
}
Alright, p in the main routine is supposed to be the first attachment,

and xPosmatter/xPosantimatter are supposed to be the second attachment.
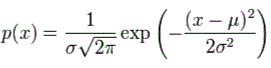
I'm getting the wrong output, so I just want to make sure I translated the math right before I go any further.