So I have a square matrix composed of several linked lists, all containing random integers.
The data diagram looks like this so far:
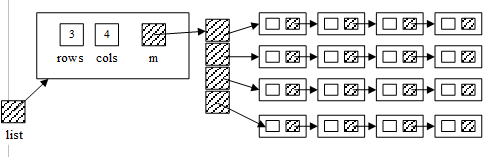
Here's all the source code for now:
Code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
#include <crtdbg.h>
typedef struct node{
int data;
struct node *link;
} NODE;
typedef struct{
int rows;
int cols;
NODE **m;
}MATRIX;
MATRIX *createMatrix(int rows, int cols);
MATRIX *buildRandomMatrix( int rows, int cols );
void printMatrix( MATRIX *matrix );
NODE *buildRandomList( int n );
void printList( NODE *pList );
void split( MATRIX *matrix ); // must be changed
int main( void )
{
// Local Definitions
MATRIX *matrix;
// Statements
matrix = buildRandomMatrix( 6, 6 );
//matrix = buildRandomMatrix( 3, 3 );
//matrix = buildRandomMatrix( 2, 2 );
//matrix = buildRandomMatrix( 1, 1 );
//matrix = buildRandomMatrix( 0, 0 );
//matrix = buildRandomMatrix( -10, -10 );
//matrix = buildRandomMatrix( 5, 6 );
if( !matrix )
printf("Invalid sizes!\n");
else
{
printf("ORIGINAL MATRIX:\n\n");
printMatrix( matrix );
split(matrix); // must be changed
printf("NEW LISTS:\n\n");
//printf( _CrtDumpMemoryLeaks() ? "Memory Leak\n" : "No Memory Leak\n");
}
system("pause");
return 0;
}
/* =======================================================
This function ...
PRE: matrix
POST: ...
*/
void split( MATRIX *matrix ) // must be changed
{
return;
}
// print up to here!
/* ********************************************************** */
/* ********************************************************** */
/* ********************************************************** */
/* =======================================================
This function allocates a header structure for a MATRIX
and a list of row NODE pointers
PRE: rows - the number of linked lists
cols - the number of elements in each linked list
POST: returns a pointer to the structure, all fields
initialized, OR NULL if not enough memory
*/
MATRIX *createMatrix(int rows, int cols)
{
MATRIX *matrix;
matrix = (MATRIX *) malloc(sizeof(MATRIX));
if( matrix )
{
matrix->rows = rows;
matrix->cols = cols;
matrix->m = (NODE **) calloc( rows, sizeof(NODE *));
if( !matrix->m ) // not enough memory
{
free(matrix);
matrix = NULL;
}
}
return matrix;
}
/* ======================================================
This function builds a matrix of rows linked lists,
each containing cols random numbers
PRE: rows - the number of linked lists
cols - the number of elements in each linked list
POST: returns a pointer to MATRIX header structure
*/
MATRIX* buildRandomMatrix( int rows, int cols )
{
// Local Definitions
MATRIX *matrix = NULL;
int r;
// Statements
srand( time(NULL) );
if ( rows > 0 && rows == cols )
{
matrix = createMatrix (rows, cols);
for( r = 0; r < rows; r++ )
matrix->m[r] = buildRandomList(cols);
}// if
return matrix;
}
/* ======================================================
This function prints a matrix of rows linked lists,
each containing cols random numbers
PRE: matrix - a pointer to the header structure of
a matrix
POST: matrix printed
*/
void printMatrix( MATRIX *matrix )
{
// Local Definitions
int r;
// Statements
for( r = 0; r < matrix->rows; r++ )
{
printf( "Row #%d: ", r + 1 );
printList(matrix->m[r]);
}
printf("\n\n\n");
return;
}
/* ======================================================
This function builds a linked list of n random numbers
PRE: n - number of nodes
POST: returns a pointer to the first node
*/
NODE *buildRandomList( int n )
{
// Local Definitions
NODE * pList;
NODE * pNew;
NODE * pPre;
int i;
// Statements
pList = NULL; //empty list
if ( n > 0 )
{
// create the first node in the list
pList = (NODE *) malloc(sizeof(NODE));
if( pList == NULL )
{
printf( "Not enough memory\n" );
exit(100);
}
pList->data = 1 + rand() % 99;
// create the rest of the nodes
pPre = pList;
for( i = 1; i < n; i++ )
{
pNew = (NODE *) malloc(sizeof(NODE));
if( pNew == NULL )
{
printf( "Not enough memory\n" );
exit(100);
}
pNew->data = 1 + rand() % 99;
pPre->link = pNew;
pPre = pNew;
}
pPre->link = NULL; // set the last node's link to NULL
}
return pList;
}
/* ======================================================
This function prints a linked list
PRE: pList - pointer to the first node in the list
POST: list printed
*/
void printList( NODE *pList )
{
NODE *pCurr;
pCurr = pList;
while( pCurr != NULL )
{
printf( "%4d", pCurr->data );
pCurr = pCurr->link;
}
printf("\n");
return;
}
I need to get it to look like this:

I'm not entirely sure how to start codewise....
I know that I must rearrange the nodes in the lists but I am not sure how to do it.
Any and all feedback is appreciated